Article Content
What is a REST API?
An API (Application Programming Interface) is an intermediate software that allows different applications to communicate and transfer data to or from each other using a set of defined rules.
In that regard, an API constitutes a set of defined rules and protocols that establish a contractual agreement between an information user (can be an actual user or another API) and information provider.
For example, in Twitter API, the user requests a twitter resource like direct messages, the API returns that information. In simple terms an API is like a Waiter(API) in a restaurant (Data provider) who takes a customer order, submits it to the kitchen(Server), and brings the food back to the customer (client).

source: google
Types of APIs
API’s are of different types including SOAP, XML-RPC, JSON-RPC and REST
1. SOAP (Simple Object Access Protocol)
SOAP is a messaging protocol primarily used to exchange data between different applications using XML (Extensible Markup Language) format over HTTP (Hypertext Transfer Protocol) .
The protocol defines the structure of the messages to be communicated between the applications and the methods of communicating.
To learn more about SOAP check on SOAP Protocol.
2. XML-RPC and JSON-RPC
These two are Remote procedure Call (RPC) protocols. They define how objects can be requested from the server and are very useful for task and actions that do not necessarily need an immediate response.
The request sent is very specific format and the returned response should be of a particular structure and include the server identification. The only difference is that XML-RPC transfers data in XML format while JSON-RPC transfers data in JSON format.
3. REST
Representational State Transfer (REST) is not necessarily a protocol but a set of design principles that an API should adhere to.
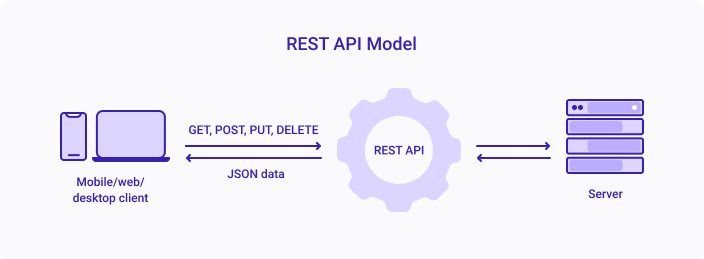
source: google
The Principles of RESTful API include:
Stateless: This means each request has to have all the information necessary for processing it. This means the server application should not store a client request data and thus server-side sessions are not required.
Uniform Interface: all requests to the API should be uniform irrespective of where it is requested from. That means the Uniform Resource Identifier (URL or URI) should be the same and thus any application should hit the same URI to get any specific data and the data is returned in the same format every time.
Caching: The resources should be cacheable on the frontend or the back end. Any response should indicate if caching is allowed for the response resources.
Client-Server Architecture: The client (Front-end) and the server (Back-end) applications should be independent of each other. The client application only knows the URI of the requested resource and the server only sends data requested and thus does not modify anything on the client application.
Layered architecture:
The client and the server should not be able to identify if they are in communication with each other directly or through intermediaries. That means responses can go through different layers of applications.
Check on REST APIs for more details on RESTful APIs.
What is FastAPI?
FAST API is a web framework for building APIs with Python 3.6+. The framework is very highly performant and fast and is based on the APIs Open Standards.
Open standards are specifications for web protocols that are maintained, created and distributed by the general international developer’s community. This means the control is by a standard body or group of organizations, it is implemented by majority of the industry and is freely available for implementation.
Examples of open standards include Open Authorization (OAuth), U2F, OpenAPI(Swagger), gRPC, GraphQL and JSON Schema.
Why Fast API?
Fast API is based on modern standard python type declarations. Type hints are enforced at runtime and errors are returned in user friendly format.
Type Hints also allows for:
1. Easy Editor Support - code completion and type checks.
2. Data Validation - automatic and clear errors on invalid data.
3. Input data conversion.
4. Output data conversion.
5. Automatic interactive API documentation.
FastAPI borrows from Starlette which is a lightweight Asynchronous Server Gateway Interface (ASGI) framework for building high performance asyncio services. This means FastAPI can create both asynchronous and synchronous applications, with a WSGI backwards-compatibility implementation and multiple servers and application frameworks.
Getting Started with FastAPI
1. Create a Virtual Environment
A virtual environment in python is an abstraction of the main python installed in your computer that is isolated in a different directory such that dependencies of different projects are separated.
Open a terminal and create a folder called fast_api_train. (I am using windows 10 and Windows Powershell as my terminal)
mkdir D:Programming/fast_api_train/
Change into the created directory.
cd D:Programming/fast_api_train/
Create a Virtual Environment named myenv.
python -m venv myenv
Activate the virtual environment.
myenv\Scripts\Activate.ps1

2. Install FastAPI
pip install fastapi
pip install "uvicorn[standard]"
Use your editor of choice and open the created fast_api_train directory as a project. In my case I am using Pycharm.
In Pycharm remember to set the Python Interpreter for your project as shown.
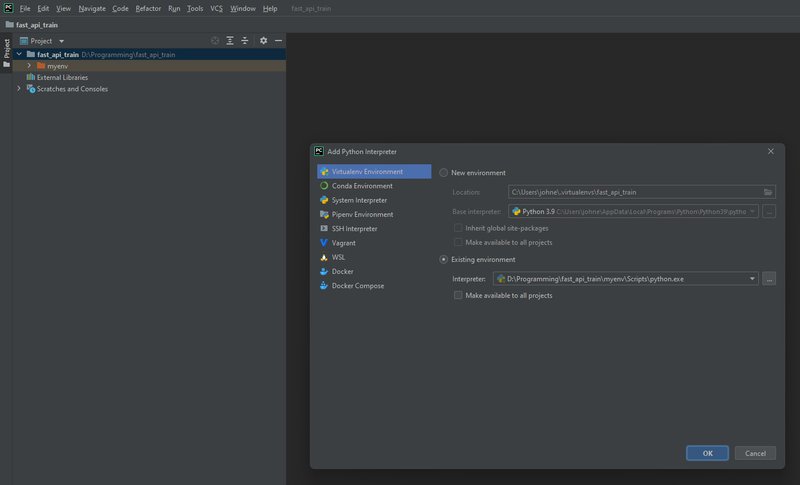
Create a main.py file and add the following code to it. We will review the code in later posts.
from fastapi import FastAPI
app = FastAPI()
fake_items_db = [{"item_name": "Foo"}, {"item_name": "Bar"}, {"item_name": "Baz"}]
@app.get("/items/")
async def read_item(skip: int = 0, limit: int = 10):
return fake_items_db[skip : skip + limit]
Run a command line script to start the unicorn server for your application
uvicorn main:app –reload
Access your running API server at:
Check your API's documentation using OpenAPI.
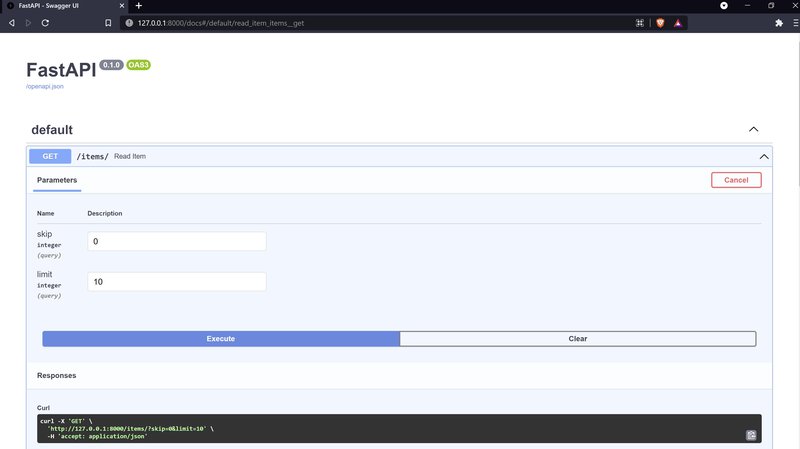
Create a requirements.txt file and add fastapi and uvicorn[standard] as the packages.

This will help when deploying your application and containerizing it with Docker.